Functions in Processing #
Processing shows how to use functions. The function drawTarget() is called 3-times, each time with different parameters setting the position and size of the rings.
Example of Functions in Processing
void setup() {
size(640, 360);
background(51);
noStroke();
noLoop();
}
void draw() {
drawTarget(width*0.25, height*0.4, 200, 4);
drawTarget(width*0.5, height*0.5, 300, 10);
drawTarget(width*0.75, height*0.3, 120, 6);
}
void drawTarget(float xloc, float yloc, int size, int num) {
float grayvalues = 255/num;
float steps = size/num;
for (int i = 0; i < num; i++) {
fill(i*grayvalues);
ellipse(xloc, yloc, size - i*steps, size - i*steps);
}
}
Functions in Godot #
To replicate the example in Godot, set the window size to 640 by 360 pixels:
Godot > menu Project > Project Settings… > tab General > Display > Window > Width: 640, Height: 360

Set the background to gray color.
Godot > menu Project > Project Settings… > tab General > Rendering > Environment > Define Clear Color
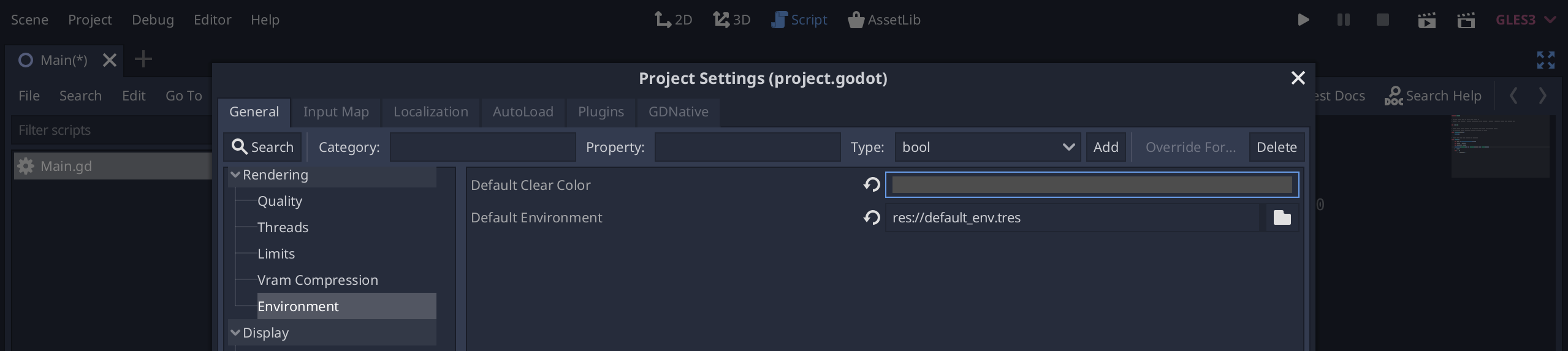
Create Node2D called Main and attach a script:
extends Node2D
# Converted from https://processing.org/examples/functions.html
#
# Set the window size to 640 by 360 pixels in
# Godot > menu Project > Project Settings... > tab General >
# Display > Window > Width: 640, Height: 360
#
# Set the background to black color in
# Godot > menu Project > Project Settings... > tab General >
# Rendering > Environment > Define Clear Color
# screen size - will be updated later on resize of the window
var width = 640
var height = 360
# Called when the node enters the scene tree for the first time.
func _ready():
# Register the size_changed event
get_tree().get_root().connect("size_changed", self, "on_size_changed")
update_width_and_height()
# Event called on window size change
func on_size_changed():
update_width_and_height()
# Read screen size and store it in the global variables
func update_width_and_height():
var size = get_viewport_rect().size
width = size.x
height = size.y
# Called automatically only once after enter scene tree.
# If re-drawing is required, call update() on this node.
func _draw():
drawTarget(width*0.25, height*0.4, 200, 4)
drawTarget(width*0.5, height*0.5, 300, 10)
drawTarget(width*0.75, height*0.3, 120, 6)
# Draw the target object
func drawTarget(xloc, yloc, size, num):
var grayvalues = 255/num
var steps = size/num
for i in range(0, num):
var c = i * grayvalues
var fillColor = color256(c, c, c)
#ellipse(xloc, yloc, size - i*steps, size - i*steps)
draw_circle(
Vector2(xloc, yloc),
(size - i*steps)/2,
fillColor)
# Helper to draw a circle
func draw_circle_arc(center, radius, angle_from, angle_to, color):
var nb_points = 64
var points_arc = PoolVector2Array()
for i in range(nb_points + 1):
var angle_point = deg2rad(angle_from + i * (angle_to-angle_from) / nb_points - 90)
points_arc.push_back(center + Vector2(cos(angle_point), sin(angle_point)) * radius)
for index_point in range(nb_points):
draw_line(points_arc[index_point], points_arc[index_point + 1], color)
# Helper to convert rgb values 0..255 to 0..1 range
func color256(r,g,b):
return Color(r/255.0, g/255.0, b/255.0)
You can download the whole zipped Godot project here: 05-Functions.zip.